2) Deploying a Sample Application Contract
Part 1: Compiling our Application Contract
Step 1) Go to https://remix.ethereum.org/
Step 2) In the File Explorer pane, click on the plus icon under the Workspaces tab to create a new workspace.
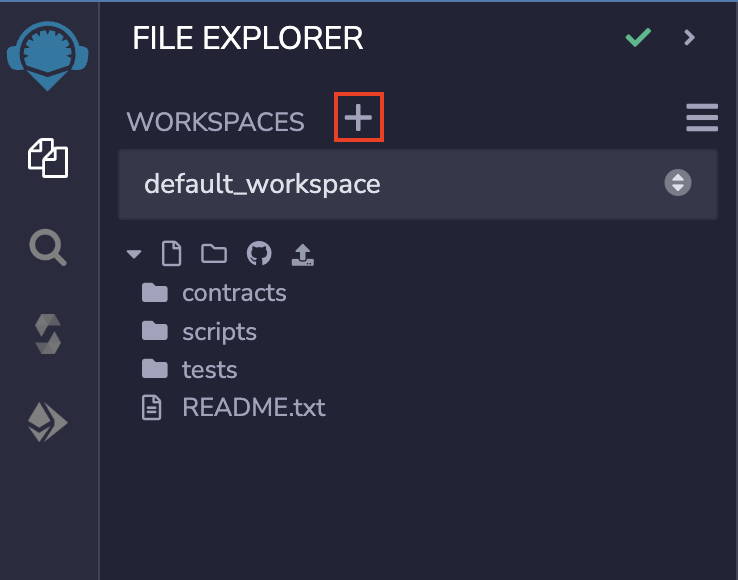
Step 3) Under the template option, choose Blank, give your workspace an appropriate name and then click on OK.
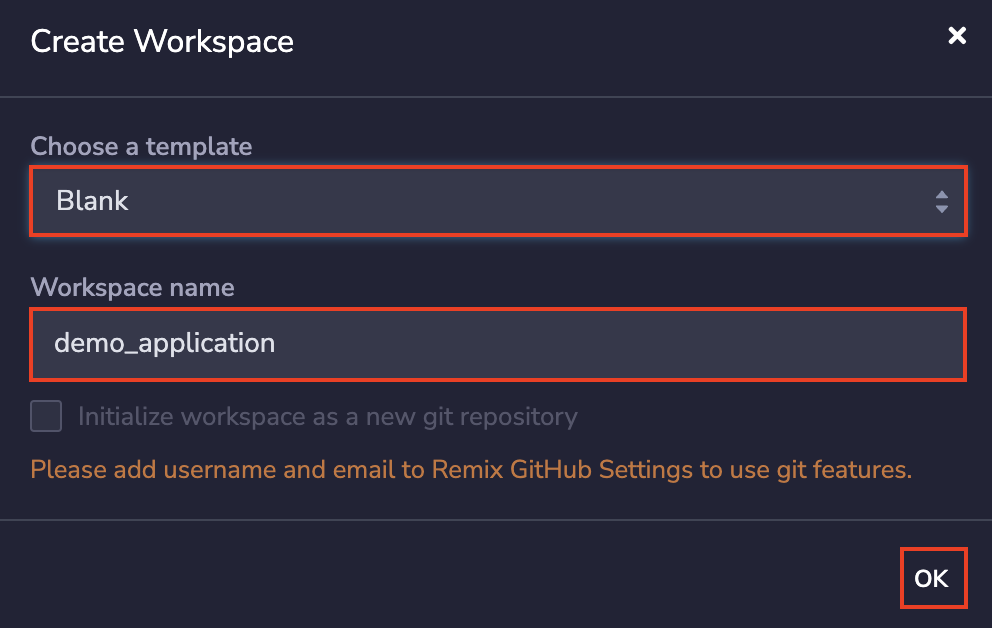
Step 4) In your new workspace, create a new file by the name of HelloRouter.sol.
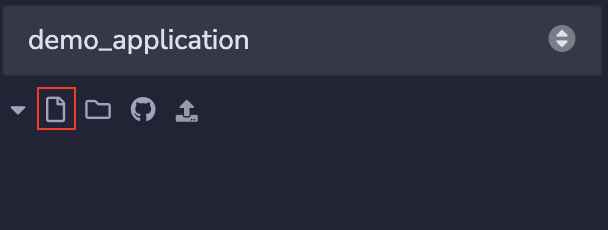
Step 5) Copy the following code and paste it into HelloRouter.sol.
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0 <0.9.0;
import "@routerprotocol/evm-gateway-contracts@1.1.11/contracts/IGateway.sol";
import "hardhat/console.sol";
contract HelloRouter {
address public owner;
IGateway public gatewayContract;
string public greeting;
error CustomError(string message);
constructor(address payable gatewayAddress, string memory _feePayer) {
owner = msg.sender;
gatewayContract = IGateway(gatewayAddress);
setDappMetadata(_feePayer);
}
function setDappMetadata(string memory FeePayer) public {
require(msg.sender == owner, "Only Owner can set fee payer");
gatewayContract.setDappMetadata(FeePayer);
}
function iSend(
string calldata destChainId,
string calldata destinationContractAddress,
string calldata str,
bytes calldata requestMetadata
) public payable {
bytes memory packet = abi.encode(str);
bytes memory requestPacket = abi.encode(destinationContractAddress, packet);
gatewayContract.iSend{ value: msg.value }(
1,
0,
string(""),
destChainId,
requestMetadata,
requestPacket
);
}
function iReceive(
string memory ,//requestSender,
bytes memory packet,
string memory //srcChainId
) external returns (string memory) {
require(msg.sender == address(gatewayContract), "only gateway");
greeting = abi.decode(
packet,
(string)
);
if (
keccak256(abi.encodePacked(greeting)) == keccak256(abi.encodePacked(""))
) {
revert CustomError("String should not be empty");
}
return greeting;
}
receive() external payable {}
}
Step 6) Click on the Solidity compiler icon (the one shaped like an S), check that your compiler version is within the versions specified in the pragma solidity statement, and click on Compile HelloRouter.sol.
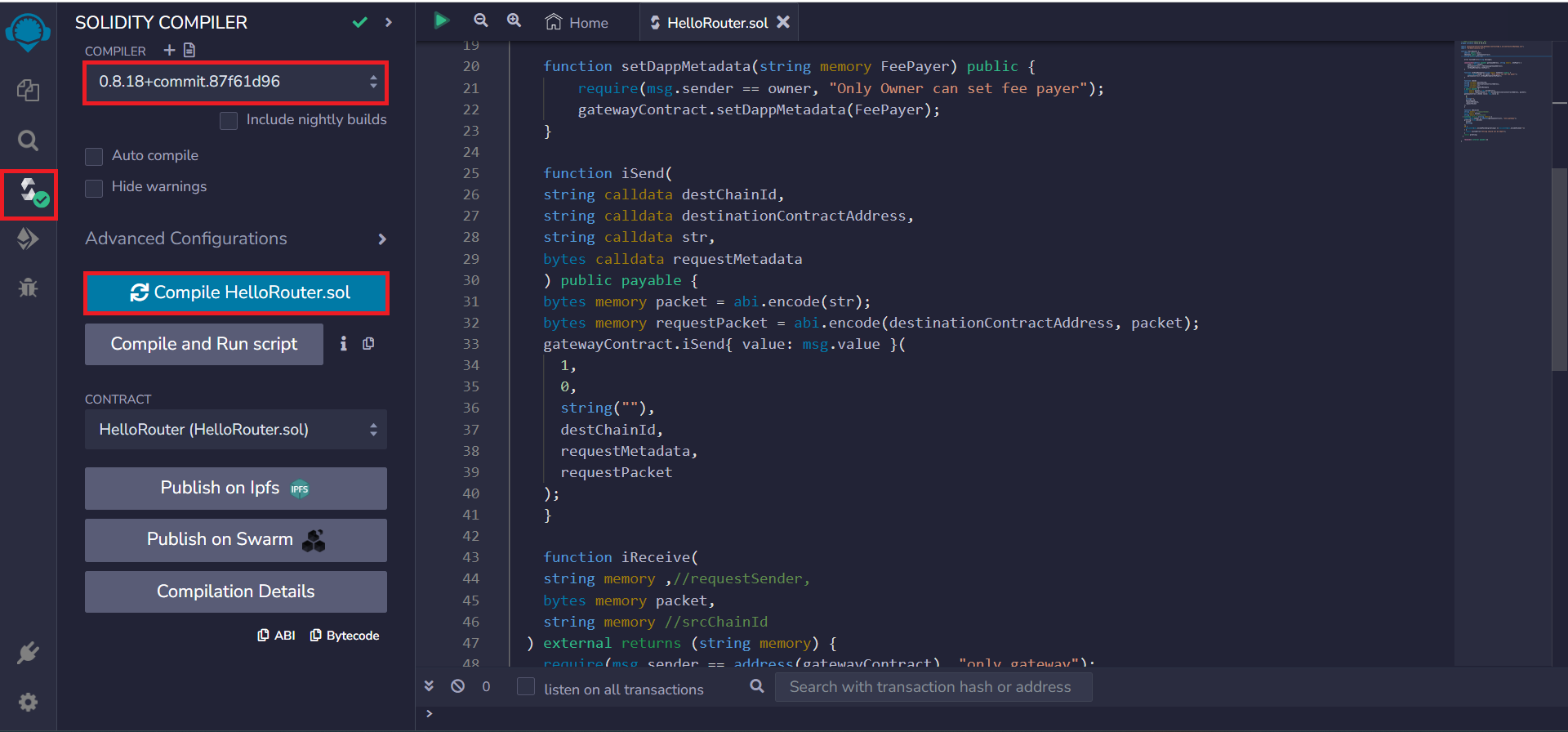
Once compiled, you should see a green tick mark over the Solidity compiler icon.
Part 2: Deploying a Compiled Contract
Step 1) Click on the Deploy & run transactions icon (the one with the Ethereum logo) and select Injected Provider - Metamask as the Environment.
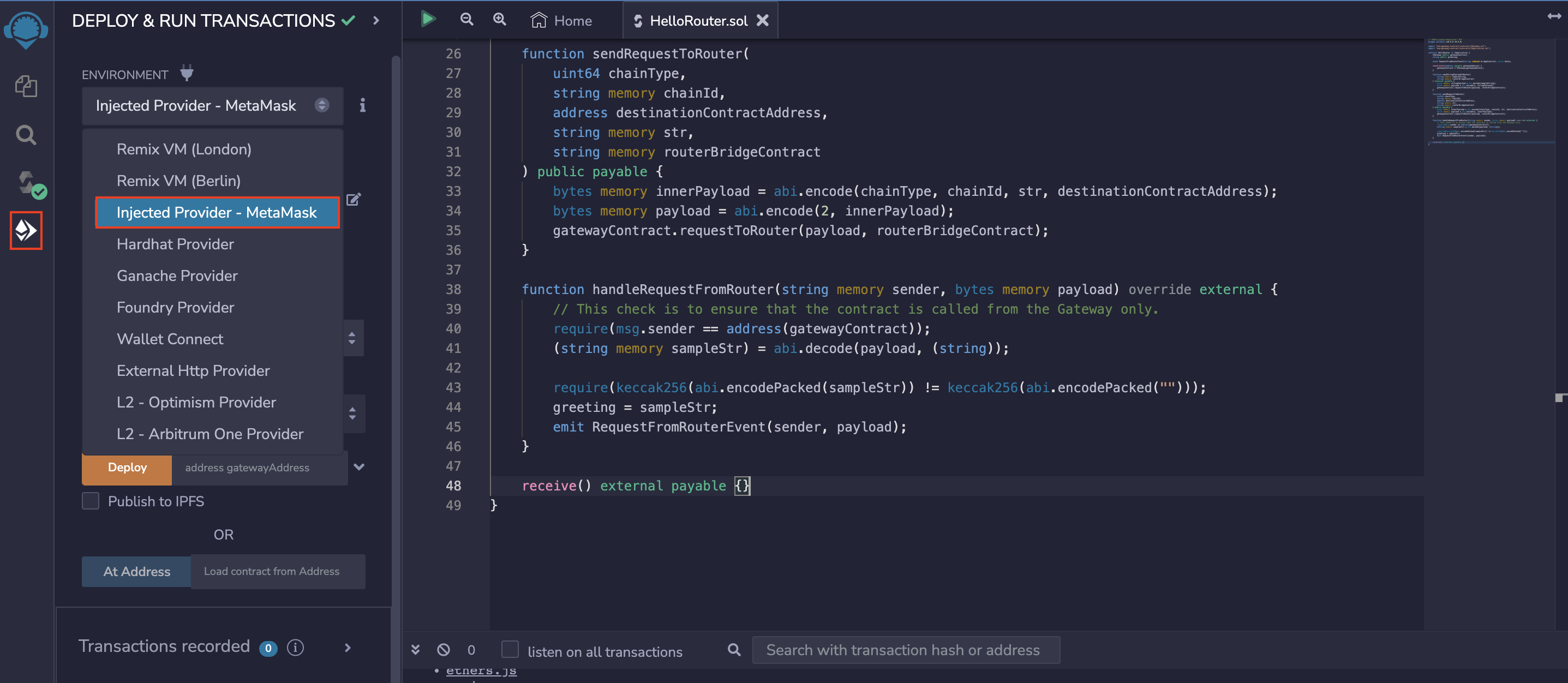
Step 2) A MetaMask window will pop up following the previous step. Connect the account that we set up in the guide given here.
Warning: Make sure you're connected to Router's EVM Testnet.
Step 3) Add the gateway address for respective chain from here, click on Deploy and sign the transaction in your MetaMask.
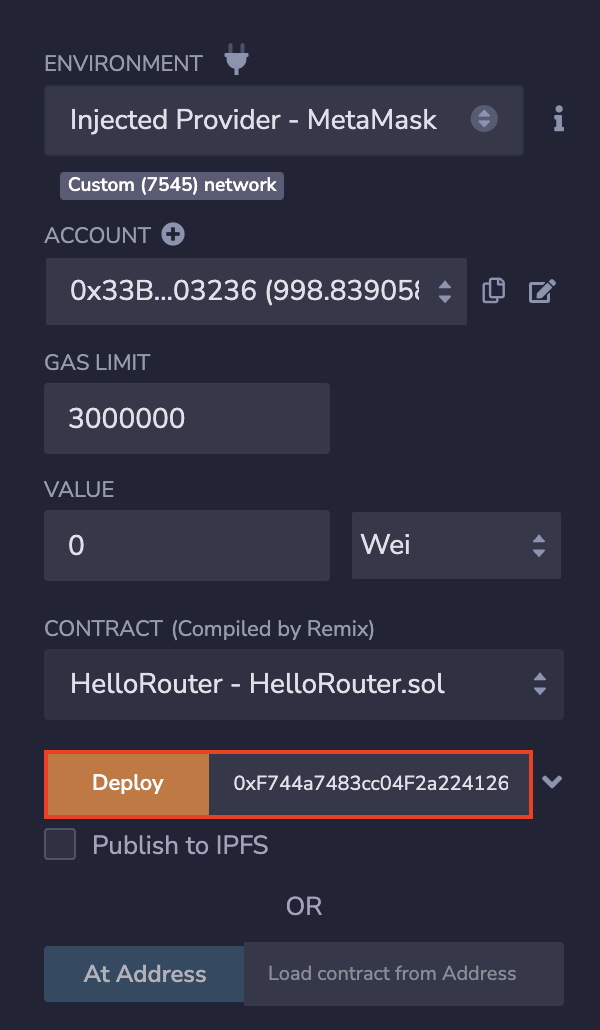
Sometimes the contract deployment fails due to low gas fees, so make sure to edit the gas fees while signing the transaction in your wallet.
